Box2D C++ tutorials - Testbed structure
Last edited: July 14 2013Chinese version -> 中文
Testbed structure
The testbed is set up in a way that allows a new test to be added efficiently. When a new test is defined, only the parts of the code which make it unique need to be written. Functions which are the same for all tests such as creating/destroying/resetting, and the control panel checkboxes and buttons, are handled by the main program code and never need to be changed.
Functions which are unique to each test - how the scene should be set up, what mouse/keyboard input should do, etc - can be specified as necessary. Before we add a test of our own, let's take a look at the life-cycle of a test.
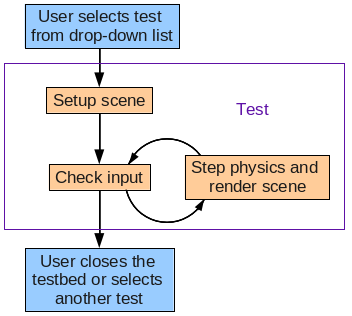
The parts in orange are where we will be adding or changing code to make our own test. To be more specific, we will create a subclass of the Test class which handles all the common features of a test, and override some functions in our subclass. Let's take a look at some virtual functions in the Test class to see what we can use.
1 2 3 4 5 | class Test ...{ virtual void Step(Settings* settings); virtual void Keyboard(unsigned char key); virtual void MouseDown(const b2Vec2& p); virtual void MouseUp(const b2Vec2& p); |
Remember that the testbed has some user input defined by default, so if this default input (mouse dragging of objects etc) is enough, then you may not need to override the input functions at all. Likewise if you are not doing anything special for rendering or physics control, you don't need to override Step() either. In fact, some of the example tests in the testbed are nothing more than a constructor to set up the scene. I told you it was simple didn't I...?
There are also some class member variables accessible from the subclass that will come in handy. These variables which keep track of the main Box2D world object which all the other thingies live in, and a body which represents the ground, a very common requirement in many simulations! We'll look at what a world and a body are a little later.
1 2 3 | protected: b2World* m_world; b2Body* m_groundBody; |