Box2D C++ tutorials - FAQ / gotchas
Last edited: February 10 2022Box2D FAQ / gotchas
Here are some questions that come up very often that don't really need a whole tutorial topic to discuss them, but still warrant mentioning somewhere to help save time and frustration when others come across them.
- Why can't I get bodies to go faster than a certain speed?
- Why is there always a small gap between bodies?
- Why do bodies lose speed after bouncing even when their restitution is set to 1?
- I'm applying an upwards force to cancel gravity, but the body still moves slowly downwards...
- Why do kinematic bodies not respond to impulses or forces?
- Why do very slow collisions cause no rebound?
- How can I get the points of fixtures to render them?
- If a ray cast starts inside a fixture, how can I find the exit point?
- How can I tell which version of Box2D I'm using?
- Why does this site mention Box2D version numbers higher than the latest available version?
- Why do I get a b2Contact for two fixtures when they're not touching?
Why can't I get bodies to go faster than a certain speed?
There is a maximum movement limit of 2.0 units per time step give in the file b2Settings.h in the source code. This might seem like a really slow speed to have as an upper limit, but this a distance per time step, not a velocity given in units per second. Units in Box2D are tuned to be meters, so at a typical framerate of 60Hz a body covering 2 units per timestep is moving at 120 m/s or 432 km/h (270 mph), which is not really that slow. You can change the value (b2_maxTranslation) if you need to, just be aware that the values in this file are tuned to work well together so you may get other problems if you change it too much.
(Note: this is a #define constant used thoughout Box2D so you'll need to recompile the library itself to make the change take effect fully.)
If you found yourself asking this question because you are using pixels as your units in the physics world, simply changing this setting to a value which allows the movement you want in pixels is probably a bad idea. It's much better to scale all the units for the physics world down by a constant ratio are so that a top speed of 2 units per timestep is more reasonable.
Why is there always a small gap between bodies?
When fixtures overlap they are pushed apart so that they don't overlap, but many cases (particularly with stacked objects) make it difficult to keep this situation stable. The box on top moves down due to gravity and touches the box underneath, then gets pushed back up, and so on, forever. Apart from looking terrible from all the jittering around, this will never stabilize and wastes computing time since the bodies will never be able to sleep.
To address this problem there is a tiny space around fixtures which is used as a buffer, in which two adjacent fixtures can agree that they are touching, but there is no overlap so no correction needs to be made - they can smile at each other and relax. This is another value in b2Settings, and while you can change it (b2_linearSlop) you should really know what you are doing before you do.
(Remember this is a #define constant used thoughout Box2D so you'll need to recompile the library itself to make the change take effect fully.)
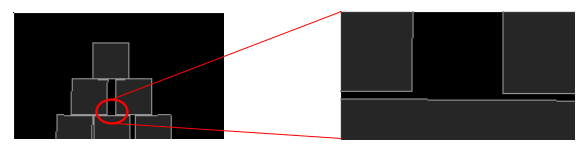
Why do bodies lose speed after bouncing even when their restitution is set to 1?
Due to the way the correcting impulses are calculated for a collision, a small amount of energy can actually be lost, especially in cases where a body collides with more than one other body at the same time. This means that a perfectly elastic collision is not guaranteed (well, the same goes for real-life situations). In very simple scenarios you may be able to adjust the velocity of the involved bodies after the collision has completed.
I'm applying an upwards force to cancel gravity, but the body still moves slowly downwards...
Check that you are applying the force before the first time step. If you have the ApplyForce call after the worlds' Step call in your main loop, the body will get one time step at normal gravity and it will have a chance to move down a tiny bit before the gravity is cancelled. Just change the order of the calls in the main loop so that the ApplyForce comes first.
Why do kinematic bodies not respond to impulses or forces?
Unlike most of the Box2D world, kinematic bodies are something we do not have in real life. Although they can move like a dynamic body, they have no mass, which means they have infinite momentum and cannot be pushed or bumped around by anything. Usually the whole point of using a kinematic body is that you don't want it to be affected by for example your player jumping on it, so it wouldn't be much use if it was affected by forces or impulses now would it? Kinematic body movements must be controlled by setting linear and angular velocities directly.
Why do very slow collisions cause no rebound?
To help keep the simulation stable very small bounces are treated as inelastic, regardless of the restitution settings you have given the fixtures. The most common case where this becomes a problem is snooker/billiards games, where even a slow collision with the side walls or the other balls should be calculated accurately. In b2Settings.h you can change the default value (b2_velocityThreshold) for the minimum velocity to consider inelastic. Changing this to a much smaller value should not usually cause any problems.
How can I get the points of fixtures to render them?
You can iterate over the points of a polygon fixture like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 | if ( fixture->GetType() == b2Shape::e_polygon ) //just to be sure { b2PolygonShape* polygonShape = (b2PolygonShape*)fixture->GetShape(); int vertexCount = polygonShape->GetVertexCount(); for (int i = 0; i < vertexCount; ++i) { //get the vertex in body coordinates b2Vec2 bcVertex = polygonShape->GetVertex( i ); //get the vertex in world coordinates b2Vec2 wcVertex = fixture->GetBody()->GetWorldPoint( bcVertex ); } } |
If a ray cast starts inside a fixture, how can I find the exit point?
Ray casts which start inside a fixture will ignore that fixture, so you will need to reverse the points and cast the ray in the other direction to find out where it would leave the fixture.
How can I tell which version of Box2D I'm using?
If you are compiling from source you can check in the file b2Settings.cpp.
If you are using a pre-compiled library you can check the values of:
1 2 3 | b2_version.major b2_version.minor b2_version.revision |
Why does this site mention Box2D version numbers higher than the latest available version?
If you are downloading the Box2D source code .zip file from the official page, you will have the most recent major release version. The major release version is usually recommended as a stable version of the source code, but it is not always the newest version in existence. Sometimes development has continued after the last major release and is stored in a 'subversion' repository for easy maintenance. This is available by 'checking out' the source code directly as shown here using a subversion client.
Chances are if you are reading this, you may not want to bother with installing a subversion client to get the latest code that way. I will keep a link here to a .zip of the latest source code so you can download it without using subversion. Please note that the latest version can contain experimental or untested features and can change at any time.
Checking latest revision...
You can check the source code changes page to see what's happening in the subversion repository.
Why do I get a b2Contact for two fixtures when they're not touching?
You may get this problem if you are looking at the list of contacts for the world with b2World::GetContactList() or for a body with b2Body::GetContactList(). The existence of a contact in these lists does not mean that the two fixtures of the contact are actually touching - it only means their AABBs are touching. If you want to know if the fixtures themselves are touching you can use:
1 | bool reallyTouching = contact->IsTouching(); |