Box2D C++ tutorials - Custom gravity
Last edited: July 14 2013Chinese version -> 中文
Specifying a different gravity for each body
A question that comes up quite often is how to make certain bodies ignore gravity, while other bodies still obey gravity. This is really easy, so now that we know about forces let's try it. Start with the same scene as in the last topic, with three identical bodies.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | //class member variable to keep track of three bodies b2Body* bodies[3]; FooTest() { //body definition b2BodyDef myBodyDef; myBodyDef.type = b2_dynamicBody; //shape definition b2PolygonShape polygonShape; polygonShape.SetAsBox(1, 1); //a 2x2 rectangle //fixture definition b2FixtureDef myFixtureDef; myFixtureDef.shape = &polygonShape; myFixtureDef.density = 1; //create identical bodies in different positions for (int i = 0; i < 3; i++) { myBodyDef.position.Set(-10+i*10, 20); bodies[i] = m_world->CreateBody(&myBodyDef); bodies[i]->CreateFixture(&myFixtureDef); } //a static floor to drop things on myBodyDef.type = b2_staticBody; myBodyDef.position.Set(0, 0); polygonShape.SetAsEdge( b2Vec2(-15,0), b2Vec2(15,0) ); m_world->CreateBody(&myBodyDef)->CreateFixture(&myFixtureDef); } |
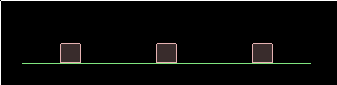
1 2 3 | //in the Step() function //cancel gravity for body 1 only bodies[1]->ApplyForce( bodies[1]->GetMass() * -m_world->GetGravity(), bodies[1]->GetWorldCenter() ); |
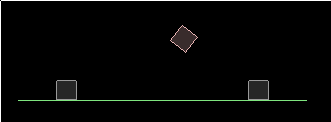
Important: This gravity-cancelling force should be applied before the first time step. If you have the ApplyForce call after the world Step call in your main loop, the body will get one time step at normal gravity and it will have a chance to move down a tiny bit before the gravity is cancelled.
Note: the above is a typical solution for the v2.1.2 release of Box2D used for these tutorials. As of v2.2.1 each body has a 'gravity scale' to strengthen or weaken the effect of the world's gravity on it. This removes the need to manually apply a force every frame. You can set this in the body definition when you create it, or use:
1 2 | //Box2D v2.2.1 onwards body->SetGravityScale(0);//cancel gravity (use -1 to reverse gravity, etc) |